[TLDR] Python doesn’t have RAII. C++ and MATLAB allows RAII. You can have a proper RAII only if destructor timing is 100% controllable by the programmer.
Python uses Context Manager (with ... as
idiom) to address the old issue of opening up a resource handler (say a file or network socket) and automatically close (free) it regardless of whether the program quit abruptly or it gracefully terminates after it’s done with the resource.
Unlike destructors in C++ and MATLAB, which registers what to do (such as closing the resource) when the program quits or right before the resource (object) is gone, Python’s Context Manager is basically rehasing the old try-block idea by creating a rigid framework around it.
It’s not that Python doesn’t know the RAII mechanism (which is much cleaner), but Python’s fundamental language design choices drove itself to a corner so it’s stuck micro-optimizing the try-except/catch-finally
approach of managing opened resourecs:
- Everything is seen as object in Python. Even integers have a ton of methods.
MATLAB and C++ treats POD, Plain Old Data, such as integers separately from classes - Python’s garbage collector controls the timing of when the destructor of any object is called (
del
merely decrement the reference count). - MATLAB’s do not garbage-collect objects so the destructor timing is guaranteed.
- C++ has no garbage collection so the destructor timing is guaranteed and managed by the programmer.
Python cannot easily exclude garbage collecting classes (which breaks RAII) because fundamentally everything are classes (dictionaries potentially with callables) in Python.
This is one of the reasons why I have a lot of respects for MATLAB for giving a lot of consideration for corner cases (like what ’empty’ means) in their language design decisions. Python has many excellent ideas but not enough thoughts was given to how these ideas interact, producing what side effects.
Pythons documentation says out loud right what it does: with ... as ...
is effectively a rigidly defined try-except-finally
block:
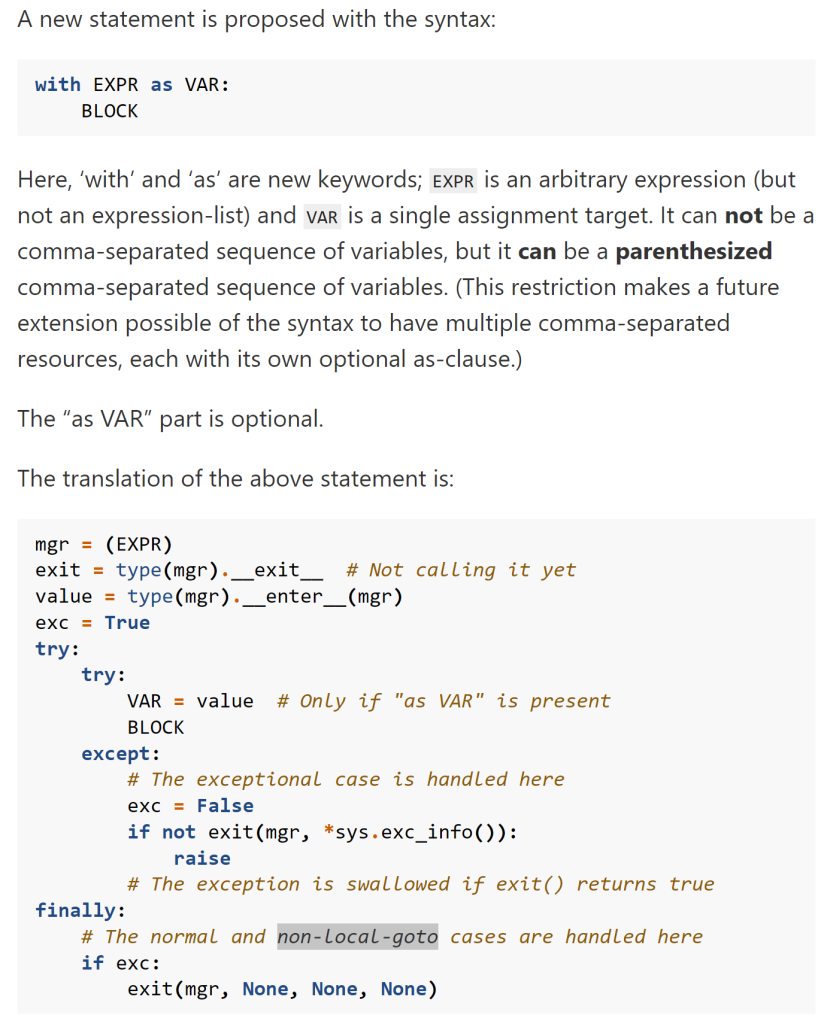
Context Manager heavily depends on resource opener function (EXPR
) to return a constructed class instance that implements __exit__
and __enter__
, so if you have a C external library imported to Python, like python-ft4222
, likely you have to write in your context manager in full when you write your wrapper.
Typically the destructor should check if the resource is already closed first, then close it if it wasn’t already closed. Take io.IOBase
as an example:
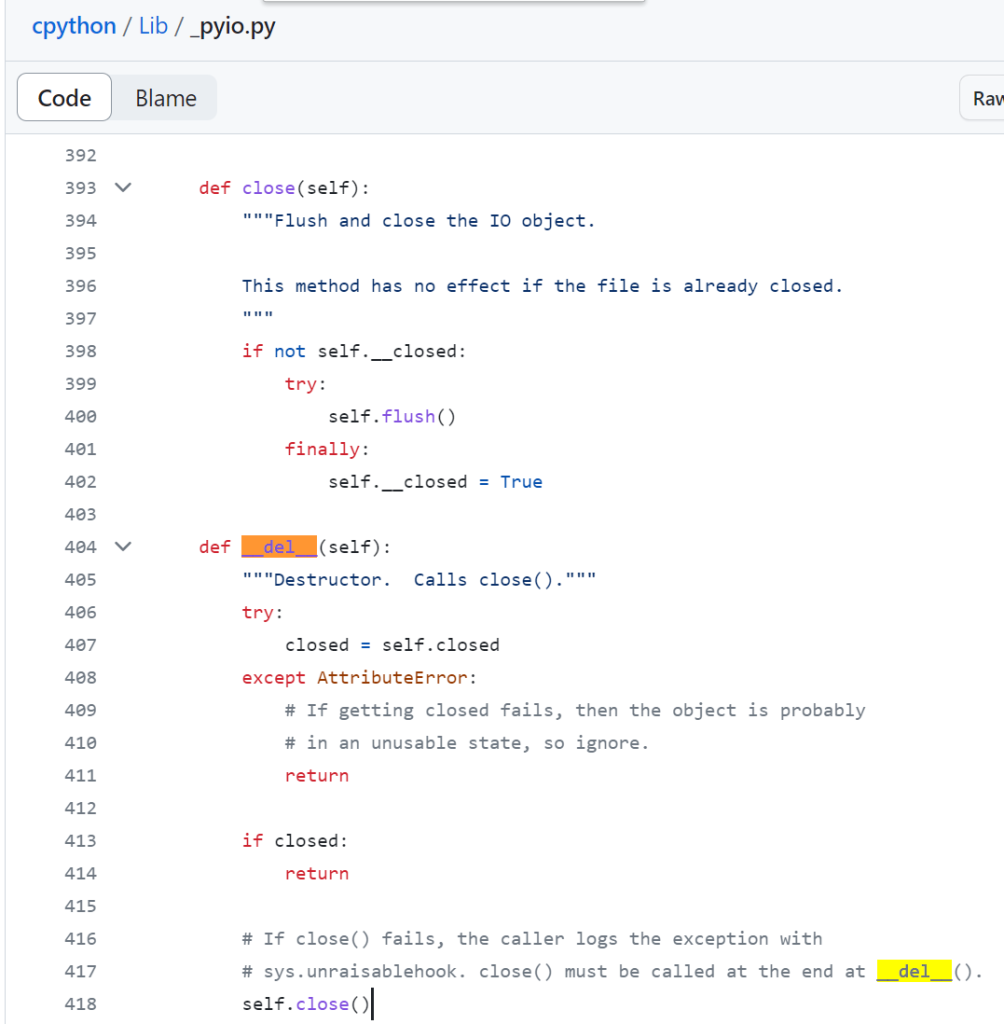
However, this is only a convenience when you are at the interpreter and can live with the destructor called with a slight delay.
To make sure your code work reliably without timing bugs, you’ll need to explicitly close it somewhere other than at a destructor or rely on object lifecycle timing. The destructor can acts as a double guard to close it again if it hasn’t, but it should not be relied on.
The with ... as
construct is extremely ugly, but it’s one of the downsides of Python that cannot be worked around easily. It also makes it difficult for users to retry acquiring a resource because one way or another retrying involves injecting the retry logic in __enter__
. It’s not that much typographic savings using with ... as
over try-except-finally
block if you don’t plan to recycle th contextmanager and the cleanup code is a one-liner.